Openpyxl is a python library, which allows us to do multiple operations read, write arithmetic operation, and different charts & graphs in Excel files. There are multiple scenarios where openpyxl would be very handy such as.
- Importing data into a database from an excel sheet.
- Exporting database data into an excel sheet.
- Appending Information to an existing sheet.
- Data validation
- Chart and bar diagram on the basis of real-time data.
Installation:
If you have anaconda installed in your system you can skip this installation process.
First of all please make sure that you have pip installed in your system. If pip is working correctly enter following command in the command line.
pip install openpyxl
Now openpyxl is installed. Let’s see how to create and write to an excel-sheet using Python.
Program to create a new excel sheet(openpyxl101.py).
#import openpyxl library and Workbook sub_module
import openpyxl
from openpyxl import Workbook
#initiate blank Workbook object
book = Workbook()
#Get workbook active sheet
sheet = book.active
#you can change sheet title using title method.
sheet.title=’quicksheet’
#We can access spreadsheet cell object
#by either [‘cell name’] or (row, column number)
sheet[‘A1’] = 56
sheet[‘A2’] = 43
sheet.cell(row=3,column=3).value=”Hello World”
book.save(‘helloworld.xlsx’)
Save it and run it through the command line or any other IDE. helloworld.xlsx file will be there in the current directory.

Output:
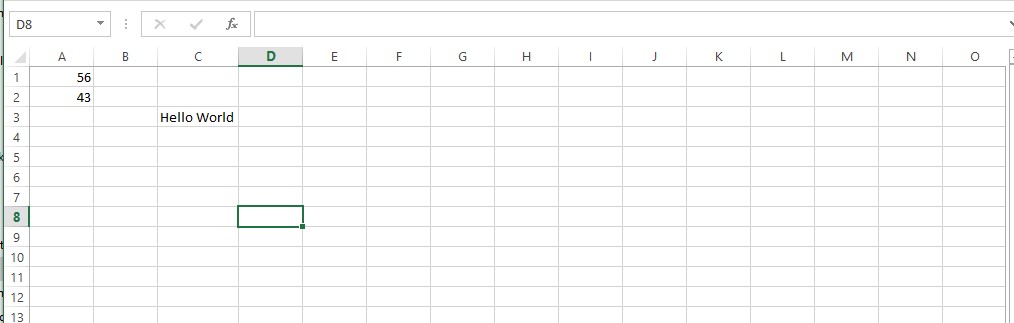