We create an instance of the BinaryCrossentropy loss function tf.keras.losses.BinaryCrossentropy(). This loss function is commonly used for binary classification tasks. It quantifies the difference between the true labels and predicted probabilities. Then, we calculate the BCE loss by calling the loss instance with the true_labels and predicted_probabilities as arguments.
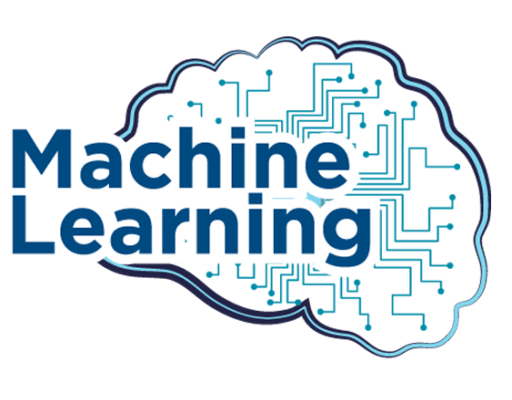